STEAMID有以下类型,通过实例函数,可以进行相互转换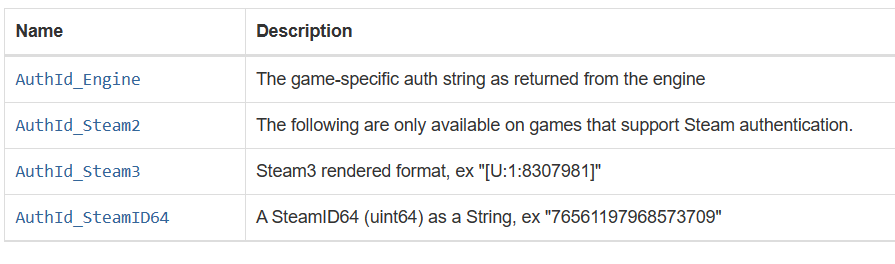
/**
* Script to format SteamID type to another type.
*
* @param input Input string where began read SteamID.
* @param output Destination string buffer.
* @param maxlength Maximum length of output string buffer.
* @param type SteamID type to format. AuthId_Steam2, AuthId_Steam3 and AuthId_SteamID64
*
* @return True when script format another SteamID. False when nothing happened.
*/
stock bool FormatSteamID(const char[] input, char[] output, int maxlength, AuthIdType type)
{
AuthIdType input_type = AuthId_Engine; // This indicate input value as ERROR
if(StrContains(input, "STEAM_", false) != -1)
{
if(strlen(input) <= 10 || input[7] != ':' || input[9] != ':')
{
return false;
}
int a = StringToInt(input[8]);
int b = StringToInt(input[10]);
if((a <= 0 && b <= 0) || a < 0 || b < 0)
return false;
input_type = AuthId_Steam2;
}
else if(StrContains(input, "[U:1:", false) != -1)
{
if(strlen(input) <= 5 || input[2] != ':' || input[4] != ':' || StringToInt(input[5]) <= 0)
{
return false;
}
input_type = AuthId_Steam3;
}
int higherbit = (1<<0)|(1<<20)|(1<<24); // 64-bit 76561197960265728 = STEAM_0:0:0
int result[2];
if(input_type == AuthId_Engine)
{
int len = strlen(input);
if(len < 17)
return false;
for(int c = 0; c < len; c++)
{
if(!IsCharNumeric(input[c]))
return false;
}
StringToInt64(input, result);
if(result[0] <= 0 ||
result[1] < higherbit)
{
return false;
}
input_type = AuthId_SteamID64;
}
switch(type)
{
case AuthId_Steam2:
{
if(input_type == AuthId_Steam2)
return false;
if(input_type == AuthId_Steam3)
{
int W = StringToInt(input[5]);
int Y = W%2;
int Z = (W-Y) / 2;
Format(output, maxlength, "STEAM_0:%i:%i", Y, Z);
return true;
}
else
{
int W = result[0];
int Y = W%2;
int Z = (W-Y) / 2;
Format(output, maxlength, "STEAM_%i:%i:%i",
result[1] >>> 24, // "Universes" ?
Y,
Z);
return true;
}
}
case AuthId_Steam3:
{
if(input_type == AuthId_Steam3)
return false;
if(input_type == AuthId_Steam2)
{
int Z = StringToInt(input[10]);
int Y = StringToInt(input[8]);
int W = Z*2+Y;
Format(output, maxlength, "[U:1:%i]", W);
return true;
}
else
{
int W = result[0];
Format(output, maxlength, "[U:1:%i]", W);
return true;
}
}
case AuthId_SteamID64:
{
if(input_type == AuthId_SteamID64)
return false;
if(input_type == AuthId_Steam2)
{
int Z = StringToInt(input[10]);
int Y = StringToInt(input[8]);
//W=Z*2+V+Y
int V = higherbit;
int W = Z*2+Y;
result[0] = W;
result[1] = V;
Int64ToString(result, output, maxlength);
return true;
}
else
{
int V = higherbit;
int W = StringToInt(input[5]);
result[0] = W;
result[1] = V;
Int64ToString(result, output, maxlength);
return true;
}
}
}
return false;
}